Hi, today i am going to tell you about how to create a SOAP Object with PHP, before that we must know about what is SOAP, a SOAP is ( SIMPLE OBJECT ACCESS PROTOCOL ) which is used for creating a Web Service, the advantage of SOAP is we can communicate with multiple and different operating system and work with multiple programming platforms on HTTP request, we can write the SOAP in XML based language, let's see how to create a simple soap server and soap client using php
What is SOAP SERVER ?
SOAP SERVER is a Constructor and provide a server to access the WSDL file and you can use this with WSDL or without WSDL service discretion, you can create the soap server in php using new keyword see this code new SoapServer("some.wsdl");
What is SOAP CLIENT ?
SOAP CLIENT is a Constructor and provide a client to access the WSDL file and you can use this with WSDL or without WSDL service discretion, you can create the soap client in php using new keyword see this code new SoapClient ("some.wsdl");
What is WSDL ?
WSDL is Web service Description Language and it is written in XML based language to describe the web services, it is a place where we write the php function names to access the SoapServer and in same wsdl file we also use the php file name with that wsdl file access the given function from php file, see the bellow XML code which i have wrote to access the users data from database
now create the Soap Server using new SoapServer('users.wsdl') and keep the wsdl file inside the constructor now we need to provide the function name to Soap Client with that the client access the data, use addFunction("getUsers") method it is predefined function of SOAP Class and inside the function assign the php function name like getUsers, now use handle() method to handle the SOAP request
- See more at: http://www.lessoncup.com/2013/12/php-soap-and-wsdl.html#sthash.O103TUeA.dpuf
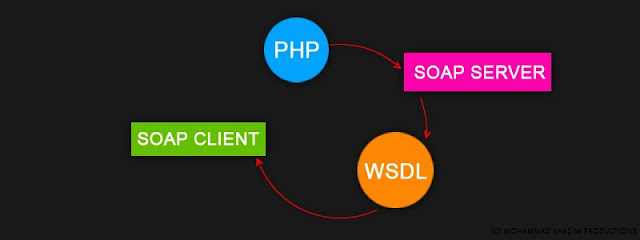
What is SOAP SERVER ?
SOAP SERVER is a Constructor and provide a server to access the WSDL file and you can use this with WSDL or without WSDL service discretion, you can create the soap server in php using new keyword see this code new SoapServer("some.wsdl");
What is SOAP CLIENT ?
SOAP CLIENT is a Constructor and provide a client to access the WSDL file and you can use this with WSDL or without WSDL service discretion, you can create the soap client in php using new keyword see this code new SoapClient ("some.wsdl");
What is WSDL ?
WSDL is Web service Description Language and it is written in XML based language to describe the web services, it is a place where we write the php function names to access the SoapServer and in same wsdl file we also use the php file name with that wsdl file access the given function from php file, see the bellow XML code which i have wrote to access the users data from database
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
|
< definitions name = 'Users' < message name = 'getUsersRequest' > < part name = 'symbol' type = 'xsd:string' /> </ message > < message name = 'getUsersResponse' > < part name = 'Result' type = 'xsd:string' /> </ message > < portType name = 'UsersPortType' > < operation name = 'getUsers' > < input message = 'tns:getUsersRequest' /> < output message = 'tns:getUsersResponse' /> </ operation > </ portType > < binding name = 'UsersBinding' type = 'tns:UsersPortType' > < operation name = 'getUsers' > < soap:operation soapAction = 'urn:localhost' /> < input > < soap:body use = 'encoded' namespace = 'urn:localhost' encodingStyle = 'http://schemas.xmlsoap.org/soap/encoding/' /> </ input > < output > < soap:body use = 'encoded' namespace = 'urn:localhost' encodingStyle = 'http://schemas.xmlsoap.org/soap/encoding/' /> </ output > </ operation > </ binding > < service name = 'UsersService' > < port name = 'UsersPort' binding = 'UsersBinding' > </ port > </ service > </ definitions > |
users.php (Creating Soap Server)
in this file create the function and write the query to fetch the users data from database and using json_encode() method convert the php array to json object, remind when ever you work with soap and wsdl you must set the cache of wsdl in server using ini_set("soap.wsdl_cache_enabled","0");now create the Soap Server using new SoapServer('users.wsdl') and keep the wsdl file inside the constructor now we need to provide the function name to Soap Client with that the client access the data, use addFunction("getUsers") method it is predefined function of SOAP Class and inside the function assign the php function name like getUsers, now use handle() method to handle the SOAP request
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
|
include ( 'db.php' ); function getUsers(){ $ss = mysql_query( "select * from users" ); $data = array (); while ( $row =mysql_fetch_array( $ss )){ $data [] = $row [ 'uid' ] . " " . $row [ 'username' ] . " " . $row [ 'email' ] . " " . $row [ 'gender' ].' '; $fabdata = json_encode( $data ); } return $fabdata ; } ini_set ( "soap.wsdl_cache_enabled" , "0" ); $server = new SoapServer( "users.wsdl" ); $server ->AddFunction( "getUsers" ); $server ->handle(); ?> |
index.php (Creating Soap Client)
here first turn off the wsdl cache, now create the soap client using new SoapClient("users.wsdl") and give the wsdl file inside the function now call the function name which is written in users.wsdl file like getUsers() now decode the JSON encoded data which is coming from function using json_decode() method, now just print or loop the elements you can able to see the result..
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
|
// turn off the WSDL cache ini_set ( "soap.wsdl_cache_enabled" , "0" ); $client = new SoapClient( "users.wsdl" ); $us = $client ->getUsers(); $users = ( array ) json_decode( $us ); while (list( $key , $val ) = each( $users )) { echo "$key => $val\n" ; } ?> |
No comments:
Post a Comment